Python有哪些语法元素:掌握基础是成功的关键
1. 变量和数据类型
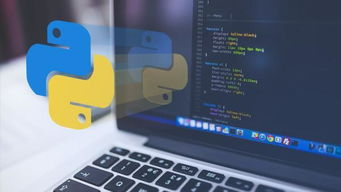
Python是一种动态类型的编程语言,这意味着在声明变量时不需要指定数据类型。变量可以存储任何类型的数据,包括整数、浮点数、字符串、列表、元组、字典等。:
```python x = 10 # 整数 y = 3.14 # 浮点数 name = "Alice" # 字符串 my_list = [1, 2, 3] # 列表 my_tuple = (4, 5) # 元组 my_dict = {"key": "value"} # 字典 ```
2. 条件语句
条件语句用于基于特定条件执行不同的代码块。Python中的条件语句主要有`if`、`elif`(else if的缩写)和`else`。:
```python age = 20 if age >= 18: print("You are an adult.") elif age >= 13: print("You are a teenager.") else: print("You are a child.") ```
3. 循环语句
循环语句允许我们重复执行一段代码。Python提供了两种类型的循环:`for`循环和`while`循环。`for`循环通常用于遍历序列(如列表、元组、字典等),而`while`循环则根据条件重复执行代码块。:
```python # for循环 for i in range(5): print(i) # while循环 count = 1 while count <= 5: print(count) count += 1 ```
4. 函数
函数是一段具有特定功能的代码块,可以接收输入(参数),并返回输出(返回值)。在Python中,使用`def`关键字定义函数。:
```python def greet(name): return f"Hello, {name}!" print(greet("Bob")) ```
5. 类和对象
Python是一种面向对象的编程语言,支持类和对象的概念。类是创建对象的蓝图,而对象是类的实例。:
```python class Car: def __init__(self, make, model): self.make = make self.model = model def display_info(self): print(f"This is a {self.make} {self.model}") my_car = Car("Toyota", "Corolla") my_car.display_info() ```
6. 模块和包
模块是包含Python代码的文件,可以包含函数、类和变量等。包是包含多个模块的目录。使用`import`语句可以导入模块或包。:
```python import math print(math.sqrt(16)) ```
7. 异常处理
异常处理用于处理程序运行中可能发生的错误。Python使用`try`、`except`、`else`和`finally`语句进行异常处理。:
```python try: result = 10 / 0 except ZeroDivisionError: print("Cannot divide by zero.") ```
8. 列表推导式
列表推导式是一种简洁的构建列表的方法,可以直接从其他列表或任何可迭代对象中创建新列表。:
```python squares = [x**2 for x in range(10)] print(squares) ```
9. 装饰器
装饰器是一种设计模式,用于修改或增强函数、方法或类的行为。在Python中,装饰器使用`@`符号和函数来实现。:
```python def my_decorator(func): def wrapper(): print("Something is happening before the function is called.") func() print("Something is happening after the function is called.") return wrapper @my_decorator def say_hello(): print("Hello!") say_hello() ```
10. 匿名函数
匿名函数是一种没有名称的函数,使用`lambda`关键字定义。它们通常用于需要函数对象的地方,但不想定义一个完整的函数。:
```python add = lambda x, y: x + y print(add(5, 3)) ```